Course pages 2012–13
Computer Graphics and Image Processing
Programming Environment for Practical Exercises
We provide a Java programming environment, Easel, that allows you to experiment with graphics algorithms, without having to worry about setting up a window.
Easel draws a pixel grid, allows you to run your algorithm on that grid (the "Run" button), and allows you to adjust the speed of execution (the slider at the top). This example is a test of twenty runs of a line drawing algorithm.
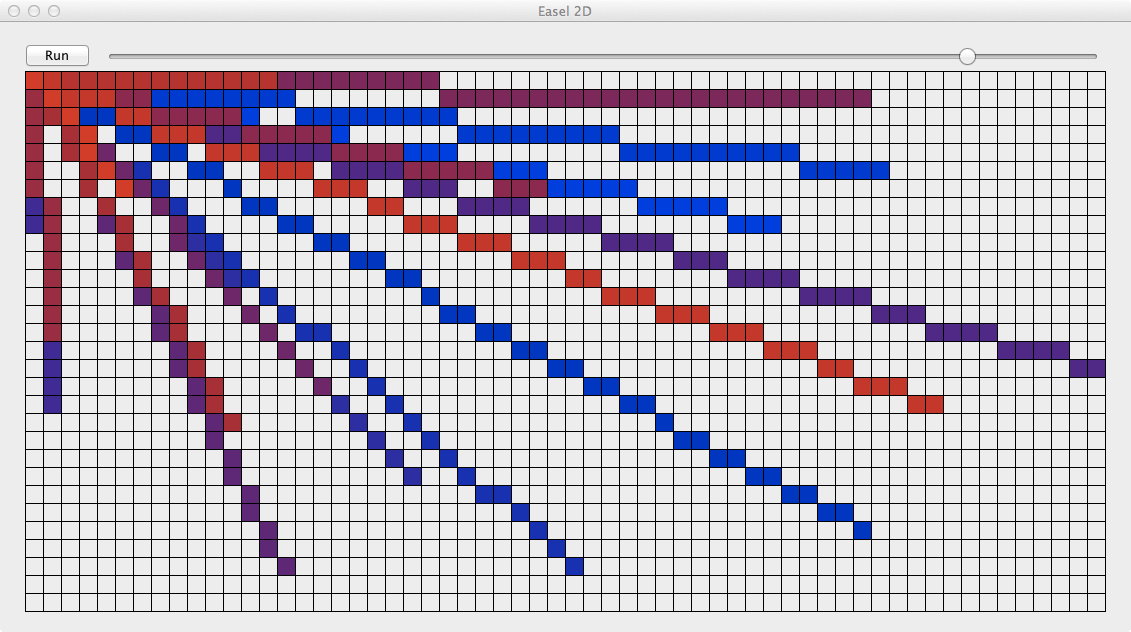
Easel downloads
- easel.jar (13.3MB, updated 7/11/12) - the Easel environment
- LineDrawer.java (3kB, updated 7/11/12) - an example of a 2D Easel program demonstrating Bresenham's line drawing algorithm
- Octahedron.java (2kB, updated 7/11/12) - an example of a 3D Easel program showing a rotating, shaded octahedron
Easel functions for 2D drawing
For 2D algorithms, Easel requires that you have a class that implements Algorithm2D. Your class's main function must call Renderer.init2D(width, height, algorithm). In the example program, this is Renderer.init2D( 40, 30, new LineDrawer() ), making a pixel grid 40 by 30 pixels, and instantiating a new LineDrawer (the class name).
Your class must include the function public void runAlgorithm( int width, int height ) which is called when the user presses the "Run" button.
To draw a pixel in the pixel grid, call the function Renderer.setPixel(x,y,red, green, blue).
Easel functions for 3D drawing
For 3D algorithms, Easel requires that you have a class that implements Algorithm3D. Your class's main function must call Renderer.init3D(algorithm). Easel sets up an OpenGL window for you.
The only required function within the class is public void renderFrame(GL2 gl) which tells Easel what to draw in that OpenGL window. You may use any OpenGL functions.
To help you with doing transformation problems, the function Renderer.drawCube(gl) draws a simple shaded cube. This is provided because drawing even the simplest shape with OpenGL requires a considerable number of function calls, as can be seen in the Octahedron.java example file.
Getting Easel to work with Eclipse
Prof. Dodgson tested the Easel resource in the Eclipse environment on both Windows 7 and Mac OS X Mountain Lion. Other Java environments will likely work but they have not been tested.
Eclipse can be downloaded free from eclipse.org For those running Ubuntu, you can simply run sudo apt-get install eclipse-platform
To connect easel.jar to a Java project in Eclipse:
- make sure that you have downloaded easel.jar and placed it in a suitable directory
- select the project in the Package Explorer pane
- menu Project->Properties
- select Java Build Path
- click Add External JARs...
- select easel.jar